之前写过一个简单String类的实现,这次再写写简单Vector类的实现,主要参照《C++ Primer(第5版)》第 13 章的一个实现,做了一些简化和修改。我们知道new有一些灵活性上的局限,它将内存分配和对象构造组合在了一起,当分配一大块内存时,我们通常计划在这块内存上按需构造对象,标准库allocator类定义在memory中,它帮助我们将内存分配和对象构造分离开来。下面是实现代码,主要使用allocator而不是new分配内存:
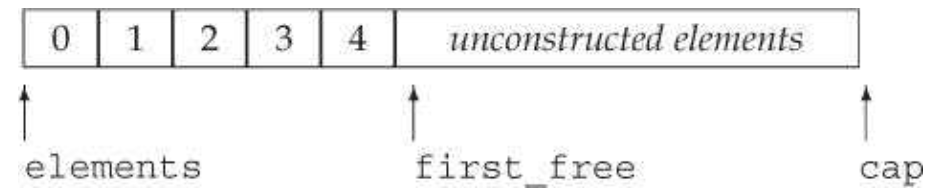
|
Personal Notes
之前写过一个简单String类的实现,这次再写写简单Vector类的实现,主要参照《C++ Primer(第5版)》第 13 章的一个实现,做了一些简化和修改。我们知道new有一些灵活性上的局限,它将内存分配和对象构造组合在了一起,当分配一大块内存时,我们通常计划在这块内存上按需构造对象,标准库allocator类定义在memory中,它帮助我们将内存分配和对象构造分离开来。下面是实现代码,主要使用allocator而不是new分配内存:
#include <memory> |